top of page
public class JackSell : Programmer, Designer {
1
2
3
4
5
6
this = jack;
jack.currentLocation = "Groningen, NL";
jack.interests = CV.interests;
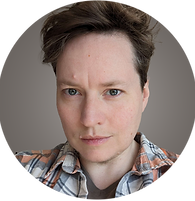
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
public class Garden Workshop: Project, Unity, VR {
[-]
#region Summary
jack.role = leadProgrammer;
jack.responsibilities = Range(state machines, ui/ux, github, inventory, physics, task delegation);
// Over the course of 9 weeks, my team of six researched, planned, designed, and developed a VR
// workshop experience for a client. The Garden Workshop is a VR game built with consideration
// for the Buitenschool Glimmen, where the player plants, grows, and collects vegetables from
// their garden.
#endregion
[-]
#region Systems Review (Code)
public void CodeReview (stateMachines) {
// My first attempt at creating a state machine for growth was based on
// using multiple scripts and abstracts. However, this proved to be
// unfriendly for the rest of the team. In order to empower my team, I
// instead built a quasi-state machine based around switch statements and
// cases per growth state.
Reflection(changes);
// Much of my time was spent making the code understandable for others,
// rather than putting that time into optimization. The systems worked,
// but doesn't allow for as much flexibility in future development and
// feature expansion.
}
public void CodeReview (inventory, recipes) {
// Process and Iteration
// To address the need for an objective in the project, I built an
// inventory system using dictionaries for items harvested. The items and
// recipes derived from scriptable objects for ease of implementation.
// This allowed "pages" in a UI clipboard per recipe that used a for loop
// to cycle through pages.
Reflection(changes);
// By building a framework with scriptable objects, my team could create
// the recipes and items themselves, streamlining the process and
// reducing my workload.
}
#endregion
51
public void DisplayMedia(Video video, List<Image> imgSet) {
video.name = "Garden Workshop Gameplay Footage"
imgSet[0].name = "RecipeUI"
imgSet[1].name = "GameScene"
imgSet[2].name = "StateChange"
imgSet[3].name = "StateChangeGrow"

Recipe UI
Using scriptable objects to reference items needed
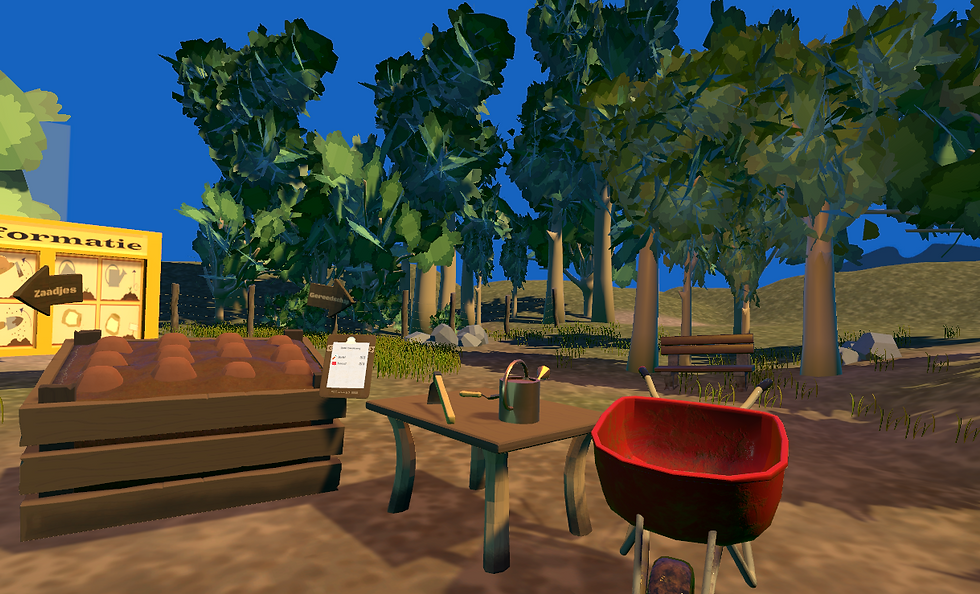
Garden Scene
Main gameplay scene
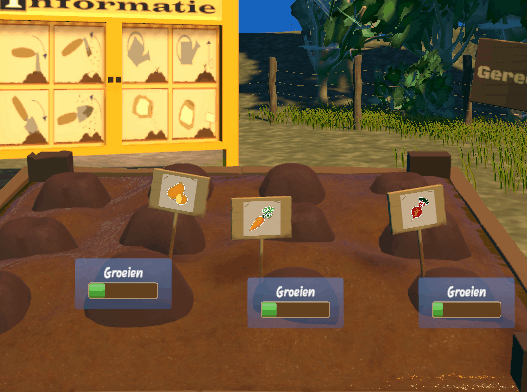
State Machine: UI
When growing, plants display a progress bar
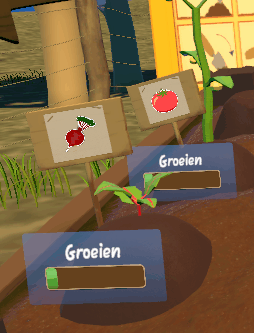
1/1
52
}
53
public class PackageDelivery : Project, Unity {
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
[-]
[-]
jack.role = soleProgrammer;
jack.responsibilities = Range(dialogue system, sprint/stamina, minor features);
// As a modified assignment, I developed systems and mechanics for another student's project. Due to
// their general lack of programming knowledge, the focus was on ease of implementation and
// accessibility.
// The requested features were interactions with NPCs including basic fetch quest functions and
// changes to the world environment based on those interactions.
#endregion
#region Summary
#region Systems Review (Code)
public void CodeReview (dialogue) {
// I chose to use scriptable objects and made an enum list in a namespace
// for dialogue. This held a list of states that the NPCs could present
// to the player, and the dialogue script pulled the appropriate
// scriptable object from a list according to the namespace. Each object
// also had a call to the next state, which progressed the NPC state when
// conditions were met.
// Quests and Dialogue were bundled into a single script to simplify
// client's usage: they only needed to attach one script to an NPC.
Reflection(changes);
// For future development, I would pursue three main things: branching
// dialogue options (player choice), randomization, and variable
// objectives.
// For dialogue loops such as Idle, implementing the option to randomize
// lines from multiple lists would be simple enough to implement.
// Instead of single objects and outcomes, each could be created as their
// own type of object. Whether a fetch quest, escort, etc, it could
// become something to slot into a “quest type” variable in the same way
// that the quest objective currently exists, then a prefab containing
// the details of that quest type. Inheritance from a parent QuestClass
// scriptable object seems like a good place to start.
// If not developing for a specific client, I would also separate the
// current script references into multiple purpose-specific scripts.
}
public void CodeReview (sprint, stamina) {
// Sprint was a simple bool tied to player speed on keypress.
// Implementing stamina introduced tracking decay over time if the
// character is sprinting. I also implemented checks for when it reached
// 0, an inverse of decay to recharge stamina, and toggling UI on/off
// depending on stamina and sprint states.
Reflection(changes);
// Further development of stamina could include impact of external
// forces, both positive and negative.
// Stamina was not a requested feature from the client. I took initiative
// to make this feature, and developed it after careful review of
// timelines for my deliverables.
}
public void CodeReview (microFeatures) {
// The client requested several small features that were quick to
// implement, including sprite swaps, animation swaps, sprite masking,
// playing a particle effect at a triggered event.
// I built several methods for each, testing iterations to see what the
// most viable solution was for the client needs.
Reflection(changes);
// These were mostly also bundled into the same NPC script as dialogue.
// This is another area where I would prefer to separate the scripts in a
// case where I was not developing for a specific client's needs.
}
#endregion
125
public void DisplayMedia(Video video) {
video.name = "Client Requested Features Demo"
126
}
178
public void DisplayMedia(Video video, List<Image> imgSet) {
video.name = "Meliae Demo Trailer"
imgSet[0].name = "Terrain Gen"
imgSet[1].name = "Terrain Falls"
imgSet[2].name = "Menu Character"
ArgumentOutOfRangeException
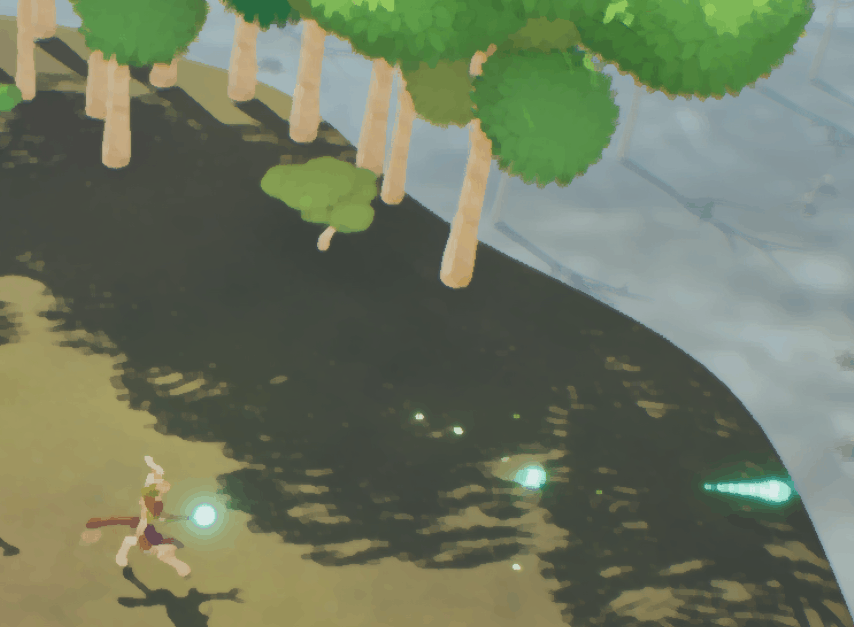
FinalBridgeSpawn
Procedural Rising Terrain
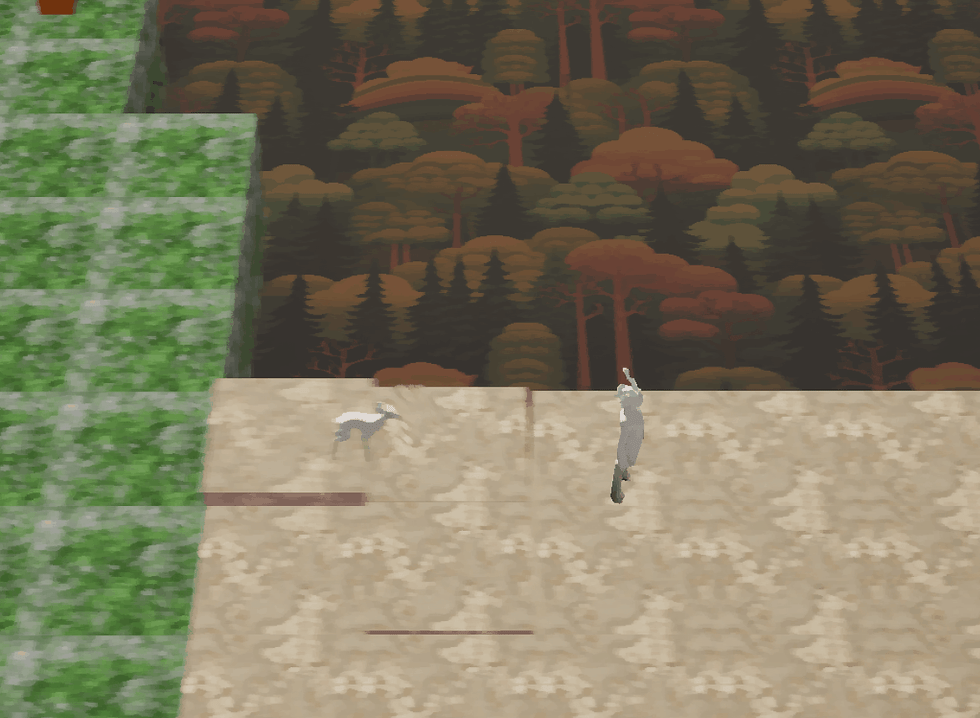
Falling Pathway
First Iteration of Falling Pathways
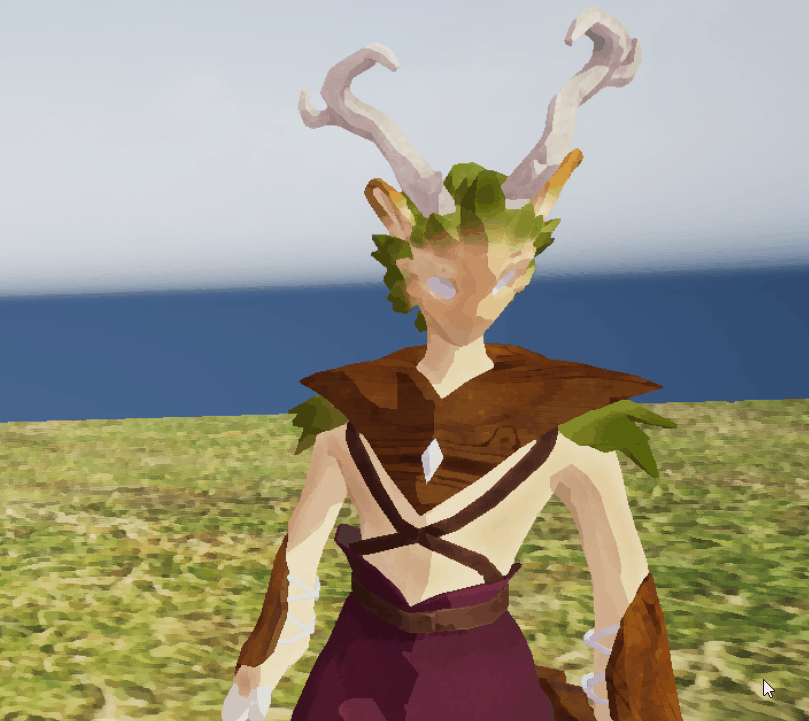
Character/Mouse Interaction
First Test of character gaze following mouse: for use in Main Menu
1/1
179
}
125
public class Meliae : Project, Unreal, EarlyDev {
[-]
#region Summary
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
jack.role = leadProgrammer;
jack.responsibilities = Range(terrain, character skills, ui, mechanics);
// Meliae is a top down bullet hell game, developed with the goal to raise awareness of deforestation
// and empower players to make change through donation and eco partnerships.
Debug.LogWarning("Showcased content in early development");
#endregion
[-]
#region Systems Review (Code)
public void CodeReview (terrainBehaviour) {
// I designed three main aspects of terrain behaviour: Level generation,
// spawning in, and falling on player collision. The terrain was built
// around the concept of blocks - being able to change terrain behavior
// (such as damage, slowdown effects) by simply changing which block is
// used.
// Falling and rising behaviour was built around use of Timelines
// triggered by player collision.
Reflection(changes);
// The systems themselves are currently in need of a redesign. I would
// change blocks to have a parent behavior and create children from the
// parent block instead of creating new blocks for each.
// Level building has gone through multiple redesigns, trying to make it
// as simple for non-programming teammates to extend the level as
// possible. However, much of it still needs to be collapsed to
// functions to avoid repeated code.
// I would also like to build branching pathways in the level generation,
// but my current blueprints do not have the flexibility and it would
// require reworking the logic.
}
public void CodeReview (playerSkills) {
// The player has a primary and secondary skill (shoot / block). I
// designed the skill system with a debug screen to test different
// variables during gameplay (fire rate, distance, projectile speed) as
// well as the logic to unlock additional skills.
Reflection(changes);
// The initial skill behaviour was attached to the player character
// blueprint, but this proved to be cumbersome with a team working on the
// project simultaneously. All skill behavior was then moved to a
// separate "manager" blueprint.
}
public void CodeReview (userInterface) {
Debug.LogWarning("Currently being developed"); }
180
public class Remember : Project, GameMaker {
225
public void DisplayMedia(Video video, List<Image> imgSet) {
video.name = "2D RPG: Remember (Gameplay)"
[-]
#region Summary
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
jack.role = soleProgrammer;
jack.responsibilities = Range(terrain, character skills, ui, mechanics);
// Remember is a 2D RPG following the journey of a ghost with amnesia, where the player's choices in
// quest resolution impact their memories of the life they lived. The demo was created to showcase
// design choices that guide a player's experience within the game.
#endregion
[-]
#region Systems Review (Code)
public void CodeReview (quests) {
// In order to further foster player connection to the game and its
// outcomes, I created a branching and multi-staged quest system.
// Quests leading to different ethical choices
// Credits screen tied to quest choices
// Dialogue branches based on character choice
// Quest interactions opening new options for players
// Timed quests
Reflection(changes);
// Remember represents early patterns of thinking. With what I know now,
// adapting Remember to a Unity project and making use of events and
// scriptable objects would make it far easier to link together different
// aspects of quests and quest tracking.
}
public void CodeReview (lighting) {
// I wanted to use lighting to direct player attention to interactable
// parts of the world as well as environmental assets to provide
// guidance. At the time this project was created, GameMaker did not have
// a lighting system.
// To overcome this lack, I set a surface of black to overlay the screen,
// then set blendmode to subtract. With this method, for objects with a
// set parent (obj_par_light), the area of the sprite is removed from the
// surface.
Reflection(changes);
// While the lighting system works, I would be interested in exploring
// other solutions in other engines. A change in perspective to 2.5D
// would also present a more dynamic feeling to lighting.
}
imgSet[3].name = "Lighting System"
imgSet[2].name = "Pathing Design"
imgSet[1].name = "Quest UI bob"
imgSet[0].name = "Ghost Fade"
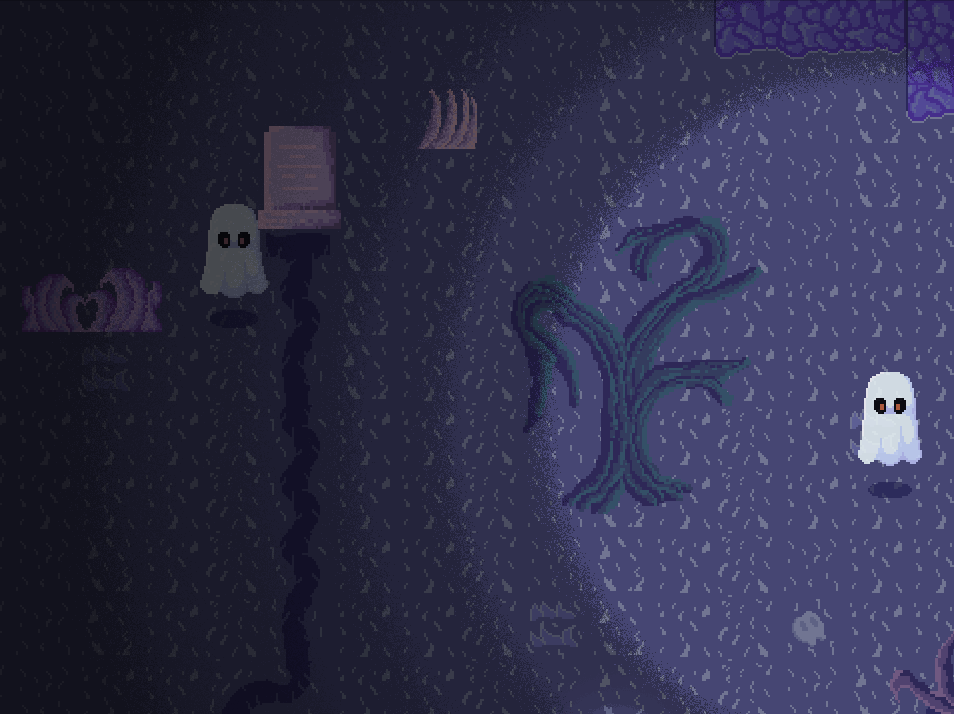
Fade Away
Non-interactable ghosts move away from the player and fade away.

Quests and Ethics
UI bob indicating a quest at this NPC
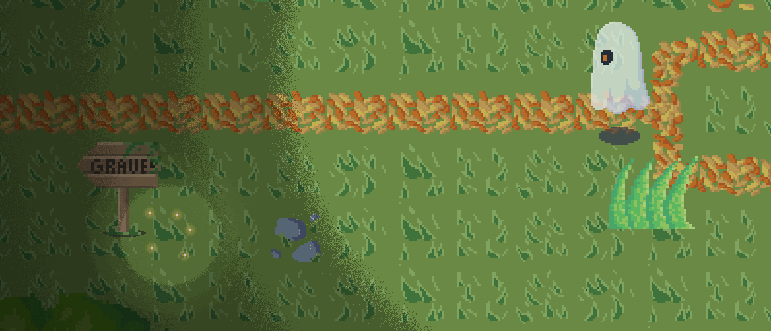
Guided Pathways
Several design choices to lead players: fireflies, contrasted pathways.
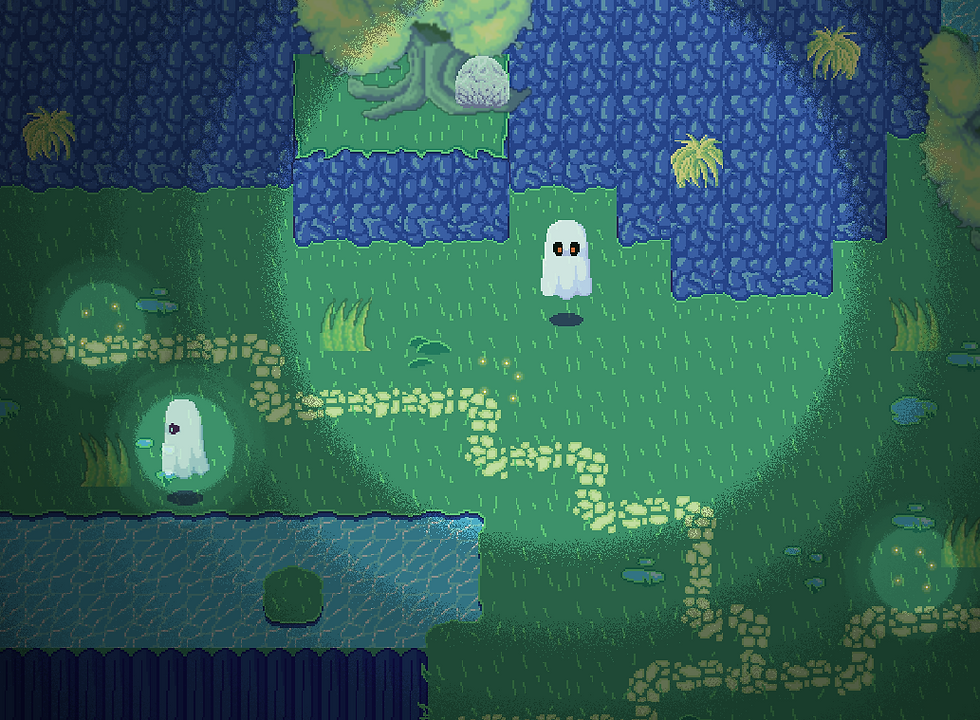
Lighting System
Drawing player attention with a lighting system (alpha punchout)
1/1
226
}
bottom of page